This article focuses on how to set up a GraphQL server from scratch, utilizing Spring Boot.
There are many different libraries with different approaches for the same. However, the library I used here has a mature Spring Boot support and is very easy to use. So this may be one of your fastest options.
Also, This article focuses only on the setup and configuration part and does not cover up basics about GraphQL. I suggest you should read official documentation to read basics and divulge more into GraphQL.
In this tutorial, we are going to use a project called graphql-tools
to integrate GraphQL
with Spring Boot. According to the project GitHub page
readme , graphql-tools
“allows you to
use the GraphQL schema language to build your graphql-java schema and allows
you to BYOO
(bring your own object) to fill in the implementations.”
It works great if you have, or want to have, plain old java objects (POJO) that define your data model. Which I do.
Note: This tutorial assumes that you are familiar with the Java programming language, the Spring Boot framework. No prior GraphQL experience is required.
Let’s get to it
Assuming you already have a spring boot project setup and ready to use.
- Add the dependency in your build.gradle file or pom.xml
- Create a GraphQL Schema file
- Define Resolver for fields in the schema
- Visualize and run through GraphIQL
1. Add the dependency in your build.gradle file or pom.xml
This Example uses Gradle so add the below dependencies to your build.gradle
file.
Keep in mind that we are using provider com.graphql-java-kickstart
instead of com.graphql-java
since the repository is moved to
avoid confusion.
... | |
dependencies { | |
... | |
compile 'com.graphql-java-kickstart:graphql-spring-boot-starter:5.0.5' | |
compile 'com.graphql-java-kickstart:graphiql-spring-boot-starter:5.0.5' | |
compile 'com.graphql-java-kickstart:graphql-java-tools:5.3.4' | |
} |
2. Create a Schema file
Create a file called schema.graphqls
in the resources
folder, with the following content:
schema { | |
query : Query | |
} | |
type Query { | |
user(id : ID) : User | |
} | |
type User { | |
id : ID ! | |
firstName : String | |
email : String | |
} |
Any files ending with the extension .graphqls within your classpath are automatically scanned and parsed.
Note: The file name is not important. However, the
extension must be .graphqls
, otherwise, the library
will not find it. The file should
also be present in the classpath of your project to be visible.
GraphQL Java Tools automatically maps fields in your GraphQL objects to those on your java objects. For most scalar fields, a POJO with fields and/or getter methods is enough to describe the data to GraphQL.
3. Define your Resolver
GraphQL Java Tools lets you register Resolvers for any type. Each field on each type is backed by the resolver.
The library will first attempt to map fields to methods on the resolver before mapping them to fields or methods on the data class.
In our case, we have declared a UserResolver that implements the GraphQLQueryResolver interface. It should also have @component or @Service Annotation.
Create a UserResolver Java class with contents :
import com.coxautodev.graphql.tools.GraphQLQueryResolver; | |
import org.springframework.stereotype.Component; | |
@Autowired | |
private UserService userService; | |
@Component | |
public class UserResolver implements GraphQLQueryResolver { | |
public User user(Long id) { | |
return userService.getUserById(id); | |
} | |
} |
Keep in mind that: a resolver method will only be called if we actually query that particular field. Otherwise, it will never be called. This is one of the main benefits of GraphQL over REST.
4. Visualize and run through GraphIql
Run your Spring boot Application! Voila!
Access the interactive graphql playground at
http://localhost:8080/graphiql
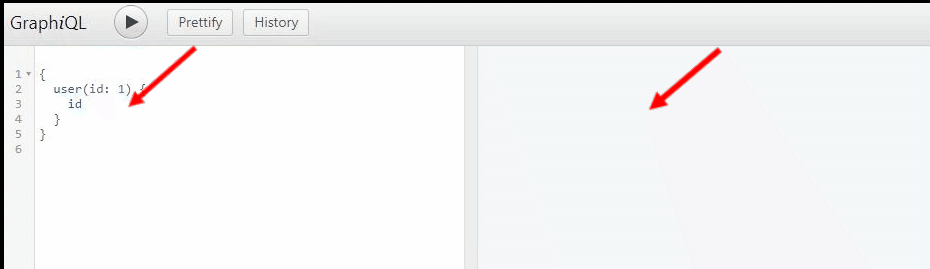